Hosting a Python web application on AWS EC2 with a MySQL database is an ideal solution for small to medium-sized applications. EC2 provides flexible virtual servers, allowing you to scale up or down as needed. In this blog, we’ll go step-by-step through deploying a Python web application using EC2 with a MySQL database.
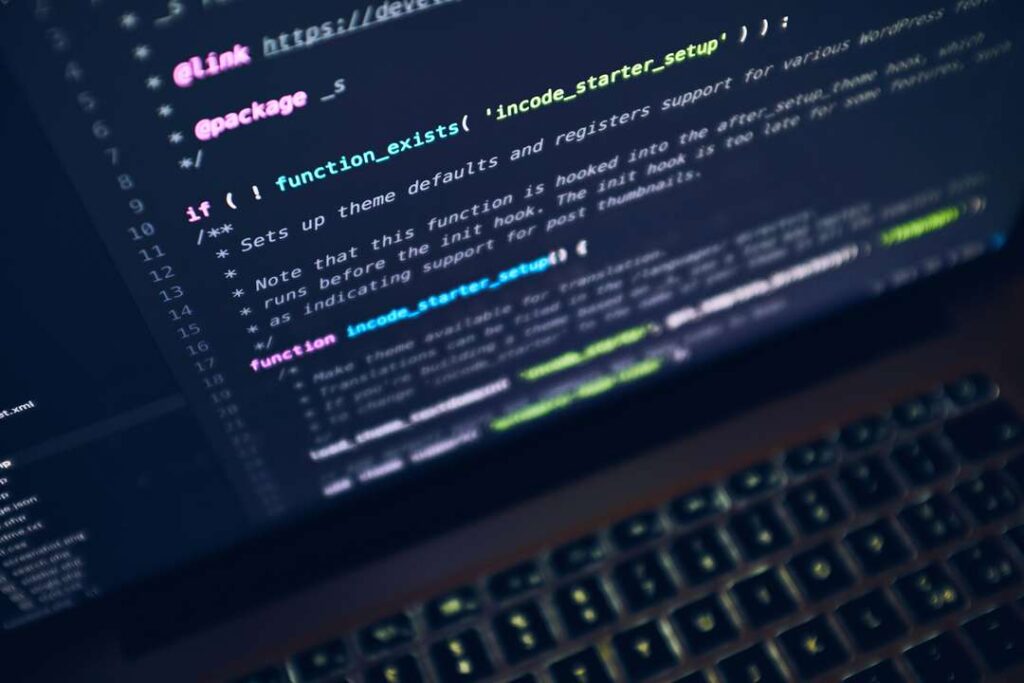
Contents
- Setting Up AWS EC2 for Python Hosting
- Configuring Security Groups for Access
- Installing Python and Required Libraries
- Setting Up and Configuring MySQL on EC2
- Deploying the Python Web Application
- Connecting Python App with MySQL
- Testing and Securing Your Application
1. Setting Up AWS EC2 for Python Hosting
Step 1: Log into AWS and Launch an EC2 Instance
- Sign into your AWS Management Console.
- Navigate to EC2 Dashboard and click Launch Instance.
- Choose an Amazon Machine Image (AMI). The Amazon Linux 2 AMI is recommended due to its compatibility and ease of use.
- Select an instance type, such as t2.micro (eligible for free tier).
- Configure the instance settings, storage, and tags as per your needs.
- In the Security Group section, create a new group or use an existing one, and make sure to allow HTTP, HTTPS, and SSH access (we’ll cover this in detail in the next section).
- Review and launch the instance. Download the private key .pem file for SSH access.
Step 2: Access the Instance via SSH
- Open a terminal and navigate to the directory where your .pem file is saved.
- Use the following command to connect, replacing your-key.pem with your actual key file and ec2-user with your EC2 user:
ssh -i "your-key.pem" ec2-user@your-ec2-public-ip
2. Configuring Security Groups for Access
- In the Security Group settings for your instance:
- Add an SSH rule on port 22 (source can be your IP for security).
- Add HTTP and HTTPS rules on ports 80 and 443 (source can be 0.0.0.0/0 for public access).
- Add a custom TCP rule to allow MySQL on port 3306 (restrict source to specific IPs or your internal IP range).
- Save changes. These rules will allow you to access your application through HTTP/HTTPS, SSH into the instance, and access the MySQL database remotely if needed.
3. Installing Python and Required Libraries
Once logged into the instance:
- Update system packages:
sudo yum update -y
- Install Python (Amazon Linux usually comes with Python installed, but you can install a specific version):
sudo yum install python3 -y
- Set up a virtual environment for your project and activate it:
python3 -m venv myenv
source myenv/bin/activate
- Install application dependencies, including Flask (or Django if you’re using it):
pip install flask pymysql
- Optionally, create a requirements.txt file to document dependencies
pip freeze > requirements.txt
4. Setting Up and Configuring MySQL on EC2
Option 1: Installing MySQL on the Same EC2 Instance
- Install MySQL server:
sudo yum install mysql-server -y
- Start the MySQL service:
sudo service mysqld start
- Secure MySQL installation by setting a root password:
sudo mysql_secure_installation
- Create a new database and user for your application:
mysql -u root -p
CREATE DATABASE myappdb;
CREATE USER 'myappuser'@'localhost' IDENTIFIED BY 'password';
GRANT ALL PRIVILEGES ON myappdb.* TO 'myappuser'@'localhost';
FLUSH PRIVILEGES;
EXIT;
Option 2: Using Amazon RDS for MySQL
You can also set up a separate MySQL instance using Amazon RDS, which manages the database for you and integrates easily with your EC2 instance.
5. Deploying the Python Web Application
- Copy your application files to the EC2 instance, either by SCP or Git.
scp -i "your-key.pem" -r /path/to/your/app ec2-user@your-ec2-public-ip:/home/ec2-user/
- Set up a simple Flask app. Here’s an example app.py file:
from flask import Flask
import pymysql
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, World! Your app is running!"
if __name__ == '__main__':
app.run(host='0.0.0.0', port=80)
- Run your application:
sudo python3 app.py
To keep your app running, consider using a process manager like Gunicorn for production, or configure Nginx to serve the app as a reverse proxy.
6. Connecting Python App with MySQL
Update your application to connect to MySQL. Here’s an example of how to do it in Flask:
import pymysql
# Connect to database
db = pymysql.connect(
host="localhost",
user="myappuser",
password="password",
database="myappdb"
)
@app.route('/data')
def get_data():
with db.cursor() as cursor:
cursor.execute("SELECT * FROM your_table")
results = cursor.fetchall()
return {"data": results}
Be sure to replace placeholders with your actual database and table names. Also, handle any potential database connection errors.
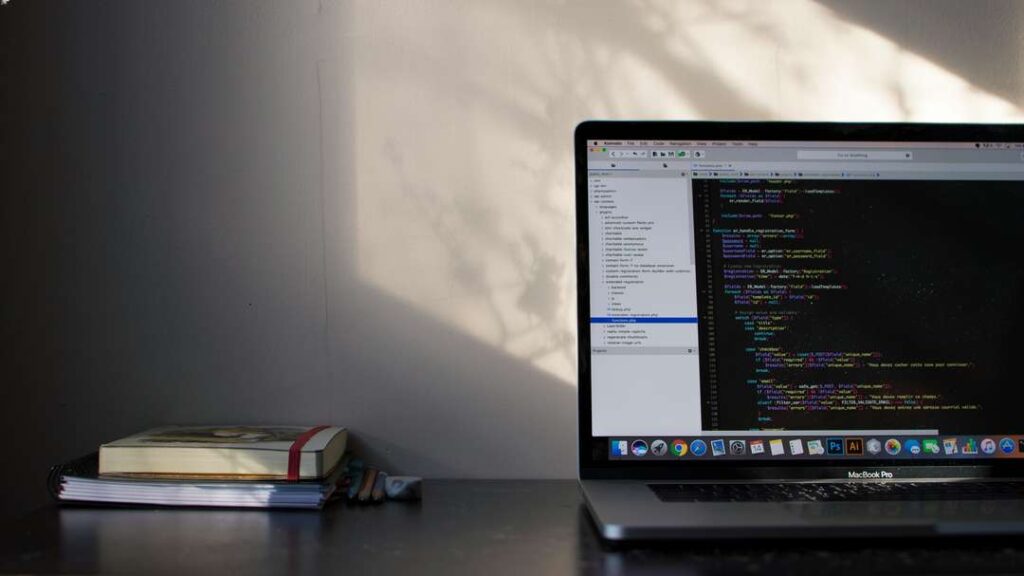
7. Testing and Securing Your Application
Testing
- Open a web browser and enter your EC2 instance’s public IP. You should see your app’s landing page (e.g., “Hello, World! Your app is running!”).
- Test other routes to ensure the MySQL connection and data retrieval work as expected.
Securing Your Application
- Set up HTTPS with a tool like Let’s Encrypt to ensure encrypted connections to your application.
- Use environment variables to store sensitive data such as database passwords.
- Enable firewall rules to limit access to specific IPs for MySQL.
- Regularly update your instance, application, and dependencies to avoid vulnerabilities.
Conclusion
Hosting a Python web application with MySQL on AWS EC2 is an accessible and flexible way to deploy and scale your projects. Following these steps, you’ve set up an EC2 instance, configured MySQL, and deployed a Python app connecting to a database. With a secure and well-tested deployment, your application is ready for real-world usage!
You can also setup with advanced tools like Docker for containerization or CI/CD pipelines for easier updates. AWS also provides scalable options if you need to add more resources to handle increased traffic in the future. Happy deploying!